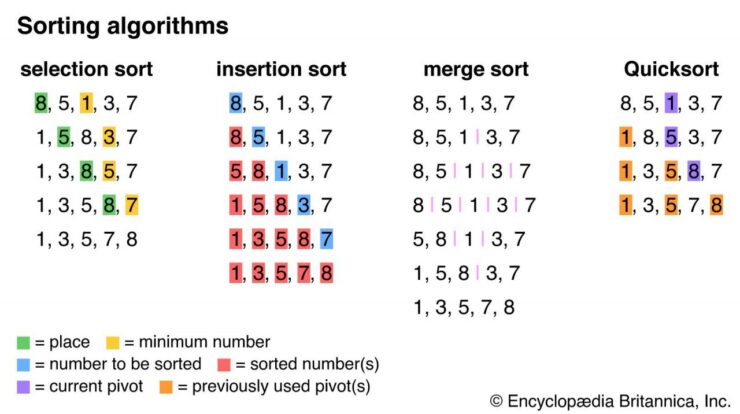
Sort definition – In the realm of data management, sorting reigns supreme as a fundamental technique for organizing and retrieving information efficiently. This comprehensive guide delves into the very essence of sorting, exploring its diverse algorithms, techniques, and applications in a manner that unravels the complexities of this essential data manipulation process.
Sorting algorithms, such as Bubble Sort, Selection Sort, Insertion Sort, Merge Sort, and Quick Sort, each employ unique strategies to arrange data in a specified order. These techniques encompass comparison-based and non-comparison-based methods, stable and unstable sorting approaches, catering to a wide range of data types and scenarios.
Sorting Algorithms: Sort Definition
Sorting algorithms are techniques used to arrange data in a specific order, typically ascending or descending. They are essential in data analysis, database management, and other applications where organized data is crucial.
Sorting algorithms work by comparing and swapping elements in an array or list until the desired order is achieved. Different sorting algorithms have different time complexities and space requirements, making them suitable for different scenarios.
Types of Sorting Algorithms
- Bubble Sort:Repeatedly compares adjacent elements and swaps them if they are out of order.
- Selection Sort:Finds the minimum element in the unsorted portion of the array and swaps it with the first unsorted element.
- Insertion Sort:Builds the sorted array one element at a time by inserting each unsorted element into its correct position.
- Merge Sort:Divides the array into smaller subarrays, sorts them recursively, and merges them back together.
- Quick Sort:Selects a pivot element, partitions the array into two subarrays based on the pivot, and recursively sorts the subarrays.
Sorting Techniques
Sorting algorithms can be categorized based on their techniques:
- Comparison-based Sorting:Compares elements to determine their order.
- Non-comparison-based Sorting:Does not compare elements but uses other properties to determine the order.
- Stable Sorting:Preserves the original order of equal elements.
- Unstable Sorting:Does not preserve the original order of equal elements.
Sorting Applications
Sorting algorithms have numerous real-world applications, including:
- Data Analysis:Organizing and analyzing large datasets to identify patterns and trends.
- Database Management:Maintaining and querying data efficiently by organizing it in sorted order.
- Computer Graphics:Sorting objects based on depth or other criteria to render scenes efficiently.
- Artificial Intelligence:Training machine learning models by sorting and analyzing data.
Performance Analysis
The performance of sorting algorithms is affected by factors such as:
- Data Size:The number of elements in the array or list.
- Data Type:The type of data being sorted (e.g., integers, strings, objects).
- Algorithm Choice:The specific sorting algorithm used.
Implementations
Sorting algorithms can be implemented in various programming languages:
- Python:Built-in sort() method and algorithms like Timsort and Merge Sort.
- Java:Arrays.sort() method and algorithms like Merge Sort, Quick Sort, and Heap Sort.
- C++:std::sort() function and algorithms like Quick Sort, Heap Sort, and Radix Sort.
- JavaScript:Array.sort() method and algorithms like Merge Sort and Quick Sort.
Advanced Sorting Techniques, Sort definition
Advanced sorting techniques include:
- Radix Sort:Sorts elements based on individual digits or bits.
- Counting Sort:Sorts elements based on the number of occurrences of each distinct element.
- Bucket Sort:Divides the array into buckets and sorts elements within each bucket.
- Heap Sort:Builds a binary heap and repeatedly extracts the maximum element to sort the array.
Data Structures for Sorting
Data structures can be used to enhance the efficiency of sorting algorithms:
- Arrays:Efficient for in-place sorting algorithms like Bubble Sort and Selection Sort.
- Linked Lists:Useful for sorting algorithms that require frequent insertions and deletions.
- Trees:Used in algorithms like Heap Sort to maintain a partially sorted order.
- Heaps:Specialized trees used in Heap Sort to efficiently extract the maximum or minimum element.
Closing Summary
The significance of sorting extends far beyond theoretical understanding, as it finds practical applications in data analysis, database management, computer graphics, artificial intelligence, and countless other domains. Understanding the nuances of sorting empowers developers and data scientists to optimize their code and extract meaningful insights from complex datasets.
Helpful Answers
What is the primary purpose of sorting?
Sorting organizes data in a specific order, facilitating efficient searching, retrieval, and analysis.
How does Bubble Sort work?
Bubble Sort repeatedly compares adjacent elements, swapping them if they are out of order, gradually “bubbling” the largest element to the end.
What is the time complexity of Merge Sort?
Merge Sort has a time complexity of O(n log n), making it one of the most efficient sorting algorithms for large datasets.